PROBLEM
Write a Python program to print all even numbers from a given numbers list and stop printing if any numbers that come after 237 in the sequence.
Sample numbers list :
numbers = 386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345, 399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217, 815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717, 958,743, 527
When solving this problem following these steps
1. Read the problem several times to understand the problem
2. Clearly write program requirements
3. Determine the inputs and outputs
3. How do you process data to get outputs from inputs?
Design your algorithm
1. The data is in a list therefore require some looping
2. During the loop we have to check if the number is even
3. We also have to check if the number is 237 to stop printing
Write the algorithm in pesudo code
Loop numbers 0 to length of list -1
if number is even (use % modular operator)
print number
if number =237 exit for loop
Draw a flow chart if the problem is complex
Only now we start thinking Python code
The solution
numbers = [386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,99, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,958,743, 527] for x in numbers: if x == 248: break elif x % 2 == 0: print(x)
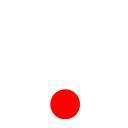