Write a Python program to print the following string in a specific format
Twinkle, twinkle, little star, How I wonder what you are! Up above the world so high, Like a diamond in the sky. Twinkle, twinkle, little star, How I wonder what you are
\n creates a new line and \t inserts a tab
print("Twinkle, twinkle, little star, \n\tHow I wonder what you are! \n\t\tUp above the world so high, \n\t\tLike a diamond in the sky. \nTwinkle, twinkle, little star, \n\tHow I wonder what you are")
1. Write a Python program which accept a number and print it.
2. Write a Python program to get the Python version you are using.
3. Write a Python program to display the current date and time.
4. Write a Python program which accept the radius of a circle from the user and compute the area. areaOfCircle=3.14 * r * r
5. Write a Python program which accept the user's first and last name and print them with a space between them.
6. Write a Python program which accepts a sequence of comma-separated numbers and generate a list with those numbers.
7. Write a Python program to accept a filename from the user print the extension of the file.
abc.txt should print txt
8. Write a Python program to display the first and last colors from the following list.
color_list = ["Red","Green","White" ,"Black"]
9. Write a Python program that accept an integer (n) and computes the value of n+nn+nnn.
if n is 5 result is 615
10. Write a Python program to test whether a number is within 0 to 100 OR 100 to 1000 or 1000 to 2000.
11. Write a Python program to calculate the sum of three given numbers, if the values are equal then return three times of their sum.
12. Write a Python program to find whether a given number is odd or even or odd.
13. Write a Python program to count from 1 to 20 in a loop.
14. Write a Python program to count from 20 to 1 in a loop.
15. Write a Python program to check whether a given value is in a group of values.
16. Write a Python program to concatenate all elements in a list into a string and return it.
18. Write a Python program that will accept the base and height of a triangle and compute the area.
19. Write a Python program that will return true if the two given integer values are equal or their sum or difference is 5.
20. Write a Python program to solve (x + y) * (x + y)
Expected Output : (4 + 3) (4+3) = 49
21. Write a Python program to compute the distance between the points (x1, y1) and (x2, y2). Use the formula SquireRoot((x2-x1) * (y2-y1))
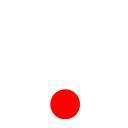