Graphics handling in Visual Basic .NET is based on GDI+ (Graphics Device Interface). A graphics device interface allows you to display graphics on a screen or a printer without having to handle the details of a specific display device.
To create a graphics object
Receive a reference to a graphics object as part of the System.Windows.Forms.PaintEventArgs in the System.Windows.Forms.Control.Paint event of a form or control.
-or-
Call the System.Windows.Forms.Control.CreateGraphics method of a control or form to obtain a reference to a System.Drawing.Graphics object that represents the drawing surface of that control or form.
-or-
Create a System.Drawing.Graphics object from any object that inherits from System.Drawing.Image. This approach is useful when you want to alter an already existing image
Private Sub Form1_Paint(ByVal sender As Object, ByVal pe As PaintEventArgs) Handles MyBase.Paint
' Declares the Graphics object and sets it to the Graphics object
' supplied in the PaintEventArgs.
Dim g As Graphics = pe.Graphics
' Insert code to paint the form here.
End Sub
Windows Forms Coordinate System
GDI+ uses three coordinate spaces: world, page, and device. World coordinates are the coordinates used to model a particular graphic world and are the coordinates you pass to methods in the .NET Framework. Page coordinates refer to the coordinate system used by a drawing surface, such as a form or control. Device coordinates are the coordinates used by the physical device being drawn on, such as a screen or sheet of paper.
When you make the call myGraphics.DrawLine(myPen, 0, 0, 160, 80), the points that you pass to the System.Drawing.Graphics.DrawLine method—(0, 0) and (160, 80)—are in the world coordinate space. Before GDI+ can draw the line on the screen, the coordinates pass through a sequence of transformations. One transformation, called the world transformation, converts world coordinates to page coordinates, and another transformation, called the page transformation, converts page coordinates to device coordinates.
Transforms and Coordinate Systems
Suppose you want to work with a coordinate system that has its origin in the body of the client area rather than the upper-left corner. Say, for example, that you want the origin to be 100 pixels from the left edge of the client area and 50 pixels from the top of the client area. The following illustration shows such a coordinate system.
The coordinates of the endpoints of your line in the three coordinate spaces are as follows:
World
(0, 0) to (160, 80)
Page
(100, 50) to (260, 130)
Device
(100, 50) to (260, 130)
The code snippet to draw the line shown in the above picture is given below:
myGraphics.TranslateTransform(100, 50)
myGraphics.DrawLine(myPen, 0, 0, 160, 80)
Drawing Text
Public Class Form1
Private Sub drawText(ByVal e As PaintEventArgs)
Dim myText As String = "Drawing Text on a Form - Visual Basic .NET 2005!"
Dim fontFamily As New FontFamily("Arial")
Dim font As New Font( _
fontFamily, _
14, _
FontStyle.Bold, _
GraphicsUnit.Point)
e.Graphics.DrawString(myText, font, Brushes.Coral, 50, 100)
End Sub
Private Sub Form1_Paint(ByVal sender As Object, ByVal e As System.Windows.Forms.PaintEventArgs) Handles Me.Paint
drawText(e)
End Sub
Private Sub Button1_Click_1(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Me.Close()
End Sub
End Class
Public Class Form1
Private Sub drawImage(ByVal e As PaintEventArgs)
Dim bmp1 As New Bitmap("C:\Discs.bmp")
e.Graphics.DrawImage(bmp1, New Point(1, 1))
End Sub
Private Sub Form1_Paint(ByVal sender As Object, ByVal e As System.Windows.Forms.PaintEventArgs) Handles Me.Paint
drawImage(e)
End Sub
End Class
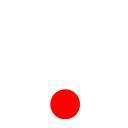