The Chat Server here is a VB.NET Console based application and is listening to the PORT 8888 for the connection request from clients . When the server gets a connection request , it add the name of the Client into a clientsList ( Here it is a Hashtable ) and create a new thread for communication with Server . When the Server get a message from any client , it select all the Clients from clientsList and send the message to all Clients ( ie we can say Broadcast ) in the clientsList . So each Client can see the message each other and they can communicate through Chat Server.
The client list we implemented here in a HashTable . The clientsList stores the Client Name ( ie the first message from Client ) and an instance of the Client Socket .
When a Chat Client connected to Server , the Server create a new Thread for communication . Here we implement a Class handleClient for handling Client as a separate Thread . The Class handleClient has a function doChat() is handling the communication between the Server side Client Socket and the incoming Client Socket.
When Server get a message from any of the currently connected Chat Client , the Server Broadcast the message to all Clients. Here we implement a function broadcast for sending messages to all Clients .
Create a new VB.NET Console based application and put the following source code into the Project.
Imports System.Net.Sockets
Imports System.Text
Module Module1
Dim clientsList As New Hashtable
Sub Main()
Dim serverSocket As New TcpListener(8888)
Dim clientSocket As TcpClient
Dim counter As Integer
serverSocket.Start()
msg("Chat Server Started ....")
counter = 0
While (True)
counter += 1
clientSocket = serverSocket.AcceptTcpClient()
Dim bytesFrom(10024) As Byte
Dim dataFromClient As String
Dim networkStream As NetworkStream = _
clientSocket.GetStream()
networkStream.Read(bytesFrom, 0, CInt(clientSocket.ReceiveBufferSize))
dataFromClient = System.Text.Encoding.ASCII.GetString(bytesFrom)
dataFromClient = _
dataFromClient.Substring(0, dataFromClient.IndexOf("$"))
clientsList(dataFromClient) = clientSocket
broadcast(dataFromClient + " Joined ", dataFromClient, False)
msg(dataFromClient + " Joined chat room ")
Dim client As New handleClinet
client.startClient(clientSocket, dataFromClient, clientsList)
End While
clientSocket.Close()
serverSocket.Stop()
msg("exit")
Console.ReadLine()
End Sub
Sub msg(ByVal mesg As String)
mesg.Trim()
Console.WriteLine(" >> " + mesg)
End Sub
Private Sub broadcast(ByVal msg As String, _
ByVal uName As String, ByVal flag As Boolean)
Dim Item As DictionaryEntry
For Each Item In clientsList
Dim broadcastSocket As TcpClient
broadcastSocket = CType(Item.Value, TcpClient)
Dim broadcastStream As NetworkStream = _
broadcastSocket.GetStream()
Dim broadcastBytes As [Byte]()
If flag = True Then
broadcastBytes = Encoding.ASCII.GetBytes(uName + " says : " + msg)
Else
broadcastBytes = Encoding.ASCII.GetBytes(msg)
End If
broadcastStream.Write(broadcastBytes, 0, broadcastBytes.Length)
broadcastStream.Flush()
Next
End Sub
Public Class handleClinet
Dim clientSocket As TcpClient
Dim clNo As String
Dim clientsList As Hashtable
Public Sub startClient(ByVal inClientSocket As TcpClient, _
ByVal clineNo As String, ByVal cList As Hashtable)
Me.clientSocket = inClientSocket
Me.clNo = clineNo
Me.clientsList = cList
Dim ctThread As Threading.Thread = New Threading.Thread(AddressOf doChat)
ctThread.Start()
End Sub
Private Sub doChat()
'Dim infiniteCounter As Integer
Dim requestCount As Integer
Dim bytesFrom(10024) As Byte
Dim dataFromClient As String
Dim sendBytes As [Byte]()
Dim serverResponse As String
Dim rCount As String
requestCount = 0
While (True)
Try
requestCount = requestCount + 1
Dim networkStream As NetworkStream = _
clientSocket.GetStream()
networkStream.Read(bytesFrom, 0, CInt(clientSocket.ReceiveBufferSize))
dataFromClient = System.Text.Encoding.ASCII.GetString(bytesFrom)
dataFromClient = _
dataFromClient.Substring(0, dataFromClient.IndexOf("$"))
msg("From client - " + clNo + " : " + dataFromClient)
rCount = Convert.ToString(requestCount)
broadcast(dataFromClient, clNo, True)
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End While
End Sub
End Class
End Module
The Chat Client is a Windows based Application and its main function is to send message to Chat Server.
Imports System.Net.Sockets
Imports System.Text
Public Class Form1
Dim clientSocket As New System.Net.Sockets.TcpClient()
Dim serverStream As NetworkStream
Dim readData As String
Dim infiniteCounter As IntegerPrivate Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim outStream As Byte() = _
System.Text.Encoding.ASCII.GetBytes(TextBox2.Text + "$")
serverStream.Write(outStream, 0, outStream.Length)
serverStream.Flush()
End SubPrivate Sub msg()
If Me.InvokeRequired Then
Me.Invoke(New MethodInvoker(AddressOf msg))
Else
TextBox1.Text = TextBox1.Text + _
Environment.NewLine + " >> " + readData
End If
End SubPrivate Sub Button2_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button2.Click
readData = "Conected to Chat Server ..."
msg()
clientSocket.Connect("127.0.0.1", 8888)
'Label1.Text = "Client Socket Program - Server Connected ..."
serverStream = clientSocket.GetStream()Dim outStream As Byte() = _
System.Text.Encoding.ASCII.GetBytes(TextBox3.Text + "$")
serverStream.Write(outStream, 0, outStream.Length)
serverStream.Flush()Dim ctThread As Threading.Thread = _
New Threading.Thread(AddressOf getMessage)
ctThread.Start()
End SubPrivate Sub getMessage()
For infiniteCounter = 1 To 2
infiniteCounter = 1
serverStream = clientSocket.GetStream()
Dim buffSize As Integer
Dim inStream(10024) As Byte
buffSize = clientSocket.ReceiveBufferSize
serverStream.Read(inStream, 0, buffSize)
Dim returndata As String = _
System.Text.Encoding.ASCII.GetString(inStream)
readData = "" + returndata
msg()
Next
End Sub
End Class
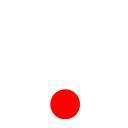