The Server Socket Program here is a VB.NET Console based Application . This program act as a Server and listening to clients request . We assign Port 8888 for the Server Socket , it is an instance of the VB.NET Class TcpListener , and call its start() method.
Dim serverSocket As New TcpListener(8888)
serverSocket.Start()
Next step we are creating an infinite loop for continuous communication to Client . When Server get the request , it reads the data from NetworkStream and also write the response to NetworkStream .
Create a new VB.NET Console Application Project and put the following source code into project.
Imports System.Net.Sockets
Imports System.Text
Module Module1
Sub Main()
Dim serverSocket As New TcpListener(8888)
Dim requestCount As Integer
Dim clientSocket As TcpClient
serverSocket.Start()
msg("Server Started")
clientSocket = serverSocket.AcceptTcpClient()
msg("Accept connection from client")
requestCount = 0
While (True)
Try
requestCount = requestCount + 1
Dim networkStream As NetworkStream = _
clientSocket.GetStream()
Dim bytesFrom(10024) As Byte
networkStream.Read(bytesFrom, 0, CInt(clientSocket.ReceiveBufferSize))
Dim dataFromClient As String = _
System.Text.Encoding.ASCII.GetString(bytesFrom)
dataFromClient = _
dataFromClient.Substring(0, dataFromClient.IndexOf("$"))
msg("Data from client - " + dataFromClient)
Dim serverResponse As String = _
"Server response " + Convert.ToString(requestCount)
Dim sendBytes As [Byte]() = _
Encoding.ASCII.GetBytes(serverResponse)
networkStream.Write(sendBytes, 0, sendBytes.Length)
networkStream.Flush()
msg(serverResponse)
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End While
clientSocket.Close()
serverSocket.Stop()
msg("exit")
Console.ReadLine()
End Sub
Sub msg(ByVal mesg As String)
mesg.Trim()
Console.WriteLine(" >> " + mesg)
End Sub
End Module
The next part of this section is Client Socket Program . After create the Client Socket Program , you should first start the Server Socket Program and then start the Client Socket Program . If you need more details about this tutorial please refer to Socket Programming Section .
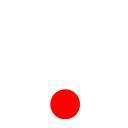