Using Directory class , we can create , delete , move etc. operations in VB.NET. Because of the static nature of Directory class , we do not have to instantiate the class. We can call the methods in the class directly from the Directory class.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object,_
ByVal e As System.EventArgs) Handles Button1.Click
If Directory.Exists("c:\testDir1") Then
'shows message if testdir1 exist
MsgBox("Directory 'testDir' Exist ")
Else
'create the directory testDir1
Directory.CreateDirectory("c:\testDir1")
MsgBox("testDir1 created ! ")
'create the directory testDir2
Directory.CreateDirectory("c:\testDir1\testDir2")
MsgBox("testDir2 created ! ")
'move the directory testDir2 as testDir in c:\
Directory.Move("c:\testDir1\testDir2", "c:\testDir")
MsgBox("testDir2 moved ")
'delete the directory testDir1
Directory.Delete("c:\testDir1")
MsgBox("testDir1 deleted ")
End If
End Sub
End Class
When you executing this program you can see , first it create directory testDir1 and then testDir2 is creating inside testDir1 , Next the program move the testDir2 to testDir . Finally it delete the directory testDir1. After the execution you can see testDir in c:\
We can create , delete , copy files etc. operations do with File class.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
If Not File.Exists("c:\testFile.txt") Then
MsgBox("FIle not exist ")
Else
File.Copy("c:\testFile.txt", "c:\testDir\testFile.txt")
MsgBox("File Copied ")
File.Delete("c:\testFile.txt")
MsgBox("file deleted ")
End If
End Sub
End Class
When you execute this program first it check whether the file exist or not , if it is not exist it shows message file does not exist, if it exist it copied the file to testDir director and it delete the file
The FileStream Class represents a File in the Computer. FileStream allows to move data to and from the stream as arrays of bytes. We operate File using FileMode in FileStream Class
Some of FileModes as Follows :
FileMode.Append : Open and append to a file , if the file does not exist , it create a new file
FileMode.Create : Create a new file , if the file exist it will append to it
FileMode.CreateNew : Create a new File , if the file exist , it throws exception
FileMode.Open : Open an existing file
How to create a file using VB.NET FileStream ?
The following example shows , how to write in a file using FileStream.
Imports System.IO
Imports System.Text
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim wFile As System.IO.FileStream
Dim byteData() As Byte
byteData = Encoding.ASCII.GetBytes("FileStream Test1")
wFile = New FileStream("streamtest.txt", FileMode.Append)
wFile.Write(byteData, 0, byteData.Length)
wFile.Close()
Catch ex As IOException
MsgBox(ex.ToString)
End Try
End Sub
End Class
When we execute the program , it create a new File and write the content to it .
Textreader and TextWriter are the another way to read and write file respectively, even though these are not stream classes. The StreamReader and StreamWriter classes are derived from TextReader and TextWriter classes respectively.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim line As String
Dim readFile As System.IO.TextReader = New _
StreamReader("C:\TextReader.txt")
While True
line = readFile.ReadLine()
If line Is Nothing Then
Exit While
Else
MsgBox(line)
End If
End While
readFile.Close()
readFile = Nothing
Catch ex As IOException
MsgBox(ex.ToString)
End Try
End Sub
End Class
When you execute this program the TextReader read the file line by line.
Textreader and TextWriter are the another way to read and write file respectively, even though these are not stream classes. The StreamReader and StreamWriter classes are derived from TextReader and TextWriter classes respectively. The following program using TextReader , Read the entire content of the file into a String
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim line As String
Dim readFile As System.IO.TextReader = New _
StreamReader("C:\Test1.txt")
line = readFile.ReadToEnd()
MsgBox(line)
readFile.Close()
readFile = Nothing
Catch ex As IOException
MsgBox(ex.ToString)
End Try
End Sub
End Class
When you execute this program , TextReader read the entire file in one stretch.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Try
Dim writeFile As System.IO.TextWriter = New _
StreamWriter("c:\textwriter.txt")
writeFile.WriteLine("shilpasayura.org")
writeFile.Flush()
writeFile.Close()
writeFile = Nothing
Catch ex As IOException
MsgBox(ex.ToString)
End Try
End Sub
End Class
When you execute this source code , you will get a file TextWriter.txt and inside it written shilpasayura.org.
BinaryReader Object works at lower level of Streams. BinaryReader is used for read premitive types as binary values in a specific encoding stream. Binaryreader Object works with Stream Objects that provide access to the underlying bytes. For creating a BinaryReader Object , you have to first create a FileStream Object and then pass BinaryReader to the constructor method .
Dim readStream As FileStream
readStream = New FileStream("c:\testBinary.dat", FileMode.Open)
Dim readBinary As New BinaryReader(readStream)
The main advantages of Binary information is that stores files as Binary format is the best practice of space utilization.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim readStream As FileStream
Dim msg As String
Try
readStream = New FileStream("c:\testBinary.dat", FileMode.Open)
Dim readBinary As New BinaryReader(readStream)
msg = readBinary.ReadString()
MsgBox(msg)
readStream.Close()
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End Sub
End Class
BinaryWriter you can use in the same way to write as binary.
Dim writeStream As FileStream
writeStream = New FileStream("c:\testBinary.dat", FileMode.Create)
Dim writeBinay As New BinaryWriter(writeStream)
The main advantages of Binary information is that it is not easily human readable and stores files as Binary format is the best practice of space utilization.
Imports System.IO
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim writeStream As FileStream
Try
writeStream = New FileStream("c:\testBinary.dat", FileMode.Create)
Dim writeBinay As New BinaryWriter(writeStream)
writeBinay.Write("This is a test for BinaryWriter !")
writeBinay.Close()
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End Sub
End Class
BinaryReader you can use in the same way to read as binary.
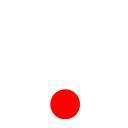