Sring Length
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
str = "This is a Test"
MsgBox(str.Length())
End Sub
End Class
When you execute this source code you will get 17 in the messagebox.
Sring Insert
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String = "This is VB.NET Test"
Dim insStr As String = "Insert "
Dim strRes As String = str.Insert(15, insStr)
MsgBox(strRes)
End Sub
End Class
When you execute this program you will get the message box showing "This is VB.NET Insert Test"
Index of
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
str = "VB.NET TOP 10 BOOKS"
MsgBox(str.IndexOf("BOOKS"))
End Sub
End Class
When you execute this program you will get the number 14 in the message box. That means the substring "BOOKS" occurred and start in the position 14.
Sring Format
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim dNum As Double
dNum = 32.123456789
MsgBox("Formated String " & String.Format("{0:n4}", dNum))
End Sub
End Class
When you run this source code yo will get "Formated String 32.1234"
Equals
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String = "Equals"
Dim str2 As String = "Equals"
If String.Equals(str1, str2) Then
MsgBox("Strings are Equal() ")
Else
MsgBox("Strings are not Equal() ")
End If
End Sub
End Class
When you run this program you will get message "Strings are Equal() "
Copyto
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String = "CopyTo() sample"
Dim chrs(5) As Char
str1.CopyTo(0, chrs, 0, 6)
MsgBox(chrs(0) + chrs(1) + chrs(2) + chrs(3) + chrs(4) + chrs(5))
End Sub
End Class
When you run this Source code you will get CopyTo in MessageBox
Contains
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String = "CopyTo() sample"
Dim chrs(5) As Char
str1.CopyTo(0, chrs, 0, 6)
MsgBox(chrs(0) + chrs(1) + chrs(2) + chrs(3) + chrs(4) + chrs(5))
End Sub
End Class
When you run this Source code you will get CopyTo in MessageBox
Contains
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
str = "VB.NET TOP 10 BOOKS"
If str.Contains("TOP") = True Then
MsgBox("The string Contains() 'TOP' ")
Else
MsgBox("The String does not Contains() 'TOP'")
End If
End Sub
End Class
When you run the program you will get the MessageBox with message "The string Contains() 'TOP' "
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
str = "VB.NET TOP 10 BOOKS"
If str.Contains("TOP") = True Then
MsgBox("The string Contains() 'TOP' ")
Else
MsgBox("The String does not Contains() 'TOP'")
End If
End Sub
End Class
When you run the program you will get the MessageBox with message "The string Contains() 'TOP' "
Compare
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String
Dim str2 As String
str1 = "vb.net"
str2 = "VB.NET"
Dim result As Integer
result = String.Compare(str1, str2)
MsgBox(result)
result = String.Compare(str1, str2, True)
MsgBox(result)
End Sub
End Class
When you run this program first u get -1 in message box and then 0
Clone
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String = "Clone() Test"
Dim clonedString As String
clonedString = str.Clone()
MsgBox(clonedString)
End Sub
End Class
When you run this program you ill get the same content of the first string "Clone() Test"
String Chars
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String = "Returned Character Chars()"
Dim singleChar As Char
singleChar = str.Chars(3)
MsgBox(singleChar)
End Sub
End Class
When you run this source code you will return a messagebox show 'u'
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String
Dim str2 As String
str1 = "vb.net"
str2 = "VB.NET"
Dim result As Integer
result = String.Compare(str1, str2)
MsgBox(result)
result = String.Compare(str1, str2, True)
MsgBox(result)
End Sub
End Class
When you run this program first u get -1 in message box and then 0
Clone
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String = "Clone() Test"
Dim clonedString As String
clonedString = str.Clone()
MsgBox(clonedString)
End Sub
End Class
When you run this program you ill get the same content of the first string "Clone() Test"
String Chars
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String = "Returned Character Chars()"
Dim singleChar As Char
singleChar = str.Chars(3)
MsgBox(singleChar)
End Sub
End Class
When you run this source code you will return a messagebox show 'u'
Substring
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
Dim retString As String
str = "This is substring test"
retString = str.Substring(8, 9)
MsgBox(retString)
End Sub
End Class
When you execute this program , its will display "subtring" in the messagebox.
Strarr
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
Dim strArr() As String
Dim count As Integer
str = "vb.net split test"
strArr = str.Split(" ")
For count = 0 To strArr.Length - 1
MsgBox(strArr(count))
Next
End Sub
End Class
When you execute this programme , you will get "vb.net" "split" "test" in separate messagebox.
Endwith
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
Dim strArr() As String
Dim count As Integer
str = "vb.net split test"
strArr = str.Split(" ")
For count = 0 To strArr.Length - 1
MsgBox(strArr(count))
Next
End Sub
End Class
When you execute this programme , you will get "vb.net" "split" "test" in separate messagebox.
Endwith
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object,
ByVal e As System.EventArgs) Handles Button1.Click
Dim str As String
str = "VB.NET TOP 10 BOOKS"
If str.EndsWith("BOOKS") = True Then
MsgBox("The String EndsWith 'BOOKS' ")
Else
MsgBox("The String does not EndsWith 'BOOKS'")
End If
End Sub
End Class
When you execute the program you will get a message box like "The String EndsWith 'BOOKS' "
Concat
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim str1 As String
Dim str2 As String
str1 = "Concat() "
str2 = "Test"
MsgBox(String.Concat(str1, str2))
End Sub
End Class
When you run this source code you will get "Concat() Test " in message box.
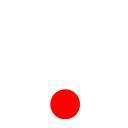