What is an Array?:
An array is a list of values referred to by a single name. Each element of an array has a unique identifying index number. In other words, an array is a collection of more than one element of data and is collectively recognized by the same variable name. The concept is similar to giving multiple menu items the same name. All the menu items execute the same event procedure when selected.
Creating One-Dimensional Arrays
What is an one-dimensional array? A segments of data that are belong to one record.
Example 1: A person's info |
|
Example 2: A list of first names |
|
To declare a one-dimensional array, you use the Dim statement with the following syntax:
Dim Name ( index ) As data type |
where:
- name is any unique name that is legitimate as a variable name.
- index is the pointer of elements in the array. The first index (element) is 0.
- data type is any data type, such as Integer, String and so on.
Just like the declaration of variables, the declaration of array variables can be placed in the general (global) declaration area or in a procedure. Arrays declared in the general declaration are form-level variables and are recognized by all procedures in the form. Arrays declared in a procedure are local (procedure-level) variables.
Examples:
Dim myFirstArray(5) As Double
The above code declares myFirstArray to be a variable containing six elements of the Double data type with subscript range of 0 to 5 as shown here: A(0) A(1) A(2) A(3) A(4) A(5)
Using One-Dimensional Arrays
Once the array has been declared, you can refer to individual elements in the array by the use of an index (subscript). For example, you declared an array as Dim arrStudents (20) As String. (*the prefix for an array is arr.) arrStudents(1) refers to the element for the first student in the arrStudents array. In this case, you don't use arrStudents(0). So, you can access it as following:
arrStudents (1) = “Steve Allen”
The above statement will assign “Steve Allen”, a text string, to the first element (1) of the arrStudents array. You can then use the element just as you would with any variable. For example, to display the name, you can code the following:
MsgBox “The first student in the array is “ & arrStudents (1)
Using Variables as the Index of an Array
An index of an array does not have be a constant. It can also be a variable or any expression that results in numeric value. So, you can code the following:
arrStudents(N) = "Jane Doe" where N is a variable acting as an index.
Based on the value of N, Jane Doe will be assigned to the corresponding element. That is, if N is 15, arrStudents(15) will be assigned with a string value Jane Doe. The ability to handle an expression as the index greatly enhances the flexibility and power of arrays, as shown below.
For k = 1 To 25
Print arrStudents (k)
Next k
It prints first 25 students from the arrStudents array
Changing Array Size
When the size of an array cannot be determined until runtime, you can use the ReDim statement to size or resize the array when the information on its size is available. To use a ReDim’ed array, you need to declare the array as Dim first. The syntax for the ReDim statement is following:
ReDim Name ( New Index Value ) As Data Typewhere:
- Name is the name of the array that you want to "re-size".
- New Index Value is the new "size" of the array.
- Data Type is any data type, such as Integer, String and so on.
The ReDim statement can be placed only within procedures; that is, you are not allowed to use the ReDim statement in the general declaration section. The following are examples of valid uses of the ReDim statement.
Dim myArray( ) As Long
ReDim myArray(10) As Long
Dim Holder( )
ReDim Holder(3 * N)
If you use a variable as a part of an expression to compute the subscript of an array, the variable must have its proper value before the ReDim statement is executed. In the preceding example, you assume proper values have been assigned to the variables EmployeeCount and N.
Passing Arrays to Procedures (or Functions)
Sometimes, an entire array needs to be passed to another procedure or function for computation or data manipulation. To pass an array to a procedure, the called procedure must be written to expect an array as its parameter. The array argument to be passed should have the variable name followed by a pair of parentheses with nothing in between. The header of the procedure being called should be written in a similar manner. The following example illustrates the syntax:
'To pass an array (e.g. Grades) from a procedure to the AveGrade function, you can code as the following:
Dim Grades(10) as Single
ClassAverage = AveGrade (Grades)
Function AveGrade (An_Array( ) As Single) As Single
statement(s)
Return An_Array(1)
End Function
A simple sample program:
Private Sub btnPassArray_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPassArray.Click
Dim theArray(5) As String
PopulateTheArray(theArray)
DisplayTheArray(theArray)
End SubPrivate Sub PopulateTheArray(ByRef theArray( ) As String)
Dim intLoopCounter As Integer
For intLoopCounter = 1 To 5
theArray(intLoopCounter) = InputBox("Enter a value for the array.")
Next intLoopCounter
End SubPrivate Sub DisplayTheArray(ByVal theArray() As String)
Dim intLoopCounter As Integer
For intLoopCounter = 1 To 5
lstArrayConten.Items.Add(theArray(intLoopCounter))
Next intLoopCounter
End Sub
Determining the Boundary of an Array
The Lbound and Ubound functions give the lower and upper boundaries of an array. For example, Lbound(An_Array) will give the low end of the subscript (index) of the array An_Array. Similarly, Ubound(An_Array) fill return the upper end of the subscript of An_Array.
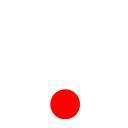