Pseudocode allows the program designer to focus on the logic of the algorithm without being distracted by details of language syntax.
The "structured" part of pseudocode is a notation for representing six specific structured programming constructs:
SEQUENCE
WHILE
IF-THEN-ELSE
REPEAT-UNTIL
FOR
CASE
Each of these constructs can be embedded inside any other to represent the logic, or flow of control in an algorithm.
It has been proven that three basic constructs for flow of control are sufficient to implement any "proper" algorithm.
SEQUENCE is a linear progression where one task is performed sequentially after another.
WHILE is a loop (repetition) with a simple conditional test at its beginning.
IF-THEN-ELSE is a decision (selection) in which a choice is made between two alternative courses of action.
REPEAT-UNTIL is a loop with a simple conditional test at the bottom.
CASE is a multiway branch (decision) based on the value of an expression. CASE is a generalization of IF-THEN-ELSE.
FOR is a "counting" loop.
Example
Brush teeth
Wash face
Comb hair
Smile in mirror
Keywords often used to indicate common input, output, and processing operations.
Input: READ, OBTAIN, GET
Output: PRINT, DISPLAY, SHOW
Compute: COMPUTE, CALCULATE, DETERMINE
Initialize: SET, INIT
Add one: INCREMENT
IF-THEN-ELSE
choice on a given Boolean condition
IF condition THEN
sequence 1
ELSE
sequence 2
ENDIF
The ELSE keyword and "sequence 2" are optional. If the condition is true, sequence 1 is performed, otherwise sequence 2 is performed.
Example
IF HoursWorked > NormalMax THEN
Display overtime message
ELSE
Display regular time message
ENDIF
WHILE
The WHILE tests at the top. The beginning and ending of the loop are indicated by two keywords WHILE and ENDWHILE. The general form is:
WHILE condition
sequence
ENDWHILE
The loop is entered only if the condition is true. The "sequence" is performed for each iteration.
At the conclusion of each iteration, the condition is evaluated and the loop continues as long as the condition is true.
Example
WHILE Population < Limit
Compute Population as Population + Births - Deaths
ENDWHILE
Example
WHILE employee.type NOT EQUAL manager AND personCount < numEmployees
INCREMENT personCount
CALL employeeList.getPerson with personCount RETURNING employee
ENDWHILE
CASE
A CASE construct indicates a multiway branch based on conditions that are mutually exclusive. Four keywords, CASE, OF, OTHERS, and ENDCASE, and conditions are used to indicate the various alternatives. The general form is:
CASE expression OF
condition 1 : sequence 1
condition 2 : sequence 2
...
condition n : sequence n
OTHERS:
default sequence
ENDCASE
The OTHERS clause with its default sequence is optional. Conditions are normally numbers or characters
REPEAT
sequence
UNTIL condition
The "sequence" in this type of loop is always performed at least once, because the test is peformed after the sequence is executed. At the conclusion of each iteration, the condition is evaluated, and the loop repeats if the condition is false. The loop terminates when the condition becomes true.
FOR
This loop is a specialized construct for iterating a specific number of times, often called a "counting" loop. Two keywords, FOR and ENDFOR are used. The general form is:
FOR iteration bounds
sequence
ENDFOR
In cases where the loop constraints can be obviously inferred it is best to describe the loop using problem domain vocabulary.
Example
FOR each month of the year (good)
FOR month = 1 to 12 (ok)
FOR each employee in the list (good)
FOR empno = 1 to listsize (ok)
NESTED CONSTRUCTS
The constructs can be embedded within each other, and this is made clear by use of indenting. Nested constructs should be clearly indented from their surrounding constructs.
Example
SET total to zero
REPEAT
READ Temperature
IF Temperature > Freezing THEN
INCREMENT total
END IF
UNTIL Temperature < zero
Print total
Loop
In the above example, the IF construct is nested within the REPEAT construct, and therefore is indented.
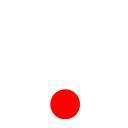