The Basics
To begin with, think of Python as pseudo-code. It’s almost true. Variables don’t have types, so you don’t have to declare them.
They appear when you assign to them, and disappear when you don’t use them anymore. Assignment is done by the = operator. Equality is tested by the == operator. You can assign several variables at once:
x,y,z = 1,2,3
first, second = second, first
a = b = 123
Blocks are indicated through indentation, and only through indentation. (No BEGIN/END or braces.) Some common control structures are:
if x < 5 or (x > 10 and x < 20):
print "The value is OK."
if x < 5 or 10 < x < 20:
print "The value is OK."
for i in [1,2,3,4,5]:
print "This is iteration number", i
x = 10
while x >= 0:
print "x is still not negative."
x = x-1
The first two examples are equivalent.
The index variable given in the for loop iterates through the elements of a list (written as in the example).
To make an “ordinary” for loop (that is, a counting loop), use the built-in function range().
# Print out the values from 0 to 99 inclusive.
for value in range(100):
print value
(The line beginning with “#” is a comment, and is ignored by the interpreter.)
Okay; now you know enough to (in theory) implement any algorithm in Python. Let’s add some basic user interaction. To get input from the user (from a text prompt), use the builtin function input.
x = input("Please enter a number: ")
print "The square of that number is", x*x
The input function displays the prompt given (which may be empty) and lets the user enter any valid Python value.
data structures. The most important ones are lists and dictionaries. Lists are written with brackets, and can (naturally) be nested:
name = ["Cleese", "John"]
x = [[1,2,3],[y,z],[[[]]]]
One of the nice things about lists is that you can access their elements separately or in groups, through indexing and slicing. Indexing is done (as in many other languages) by appending the index in brackets to the list. (Note that the first element has index 0).
print name[1], name[0] # Prints "John Cleese"
name[0] = "Smith"
Slicing is almost like indexing, except that you indicate both the start and stop index of the result, with a colon (“:“) separating them:
x = ["spam","spam","spam","spam","spam","eggs","and","spam"]
print x[5:7] # Prints the list ["eggs","and"]
every element has a key, or a “name” which is used to look up the element just like in a real dictionary. A couple of example dictionaries:
{ "Alice" : 23452532, "Boris" : 252336,
"Clarice" : 2352525, "Doris" : 23624643}
person = { 'first name': "Robin", 'last name': "Hood",
'occupation': "Scoundrel" }
Now, to get person‘s occupation, we use the expression person["occupation"]. If we wanted to change his last name, we could write:
person['last name'] = "of Locksley"
Like lists, dictionaries can hold other dictionaries. Or lists, for that matter. And naturally lists can hold dictionaries too.
Functions
Next step: Abstraction. We want to give a name to a piece of code, and call it with a couple of parameters. In other words — we want to define a function (or “procedure”). That’s easy. Use the keyword def like this:
def square(x):
return x*x
print square(2) # Prints out 4
For those of you who understand it: When you pass a parameter to a function, you bind the parameter to the value, thus creating a new reference. If you change the “contents” of this parameter name (i.e. rebind it) that won’t affect the original. This works just like in Java, for instance. Let’s take a look at an example:
def change(some_list):
some_list[1] = 4
x = [1,2,3]
change(x)
print x # Prints out [1,4,3]
As you can see, it is the original list that is passed in, and if the function changes it, these changes carry over to the place where the function was called. Note, however the behaviour in the following example:
def nochange(x):
x = 0
y = 1
nochange(y)
print y # Prints out 1
Why is there no change now? Because we don’t change the value! The value that is passed in is the number 1 — we can’t change a number in the same way that we change a list. The number 1 is (and will always be) the number 1. What we did do is change the contents of the local variable (parameter) x, and this does not carry over to the environment.
For those of you who didn’t understand this: Don’t worry — it’s pretty easy if you don’t think too much about it :)
Python has all kinds of nifty things like named arguments and default arguments and can handle a variable number of arguments to a single function. For more info on this, see the Python tutorial’s section 4.7.
If you know how to use functions in general, this is basically what you need to know about them in Python. (Oh, yes… The return keyword halts the execution of the function and returns the value given.) One thing that might be useful to know, however, is that functions are values in Python. So if you have a function like square, you could do something like:
queeble = square
print queeble(2) # Prints out 4
To call a function without arguments you must remember to write doit() and not doit. The latter, as shown, only returns the function itself, as a value. (This goes for methods in objects too… See below.)
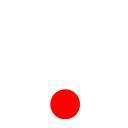