Python is an easy to learn, powerful programming language. Phython allows students to focus on problem-solving skills and algorithm development and to write simple programs to learn programming.
As well as It has efficient high-level data structures and a simple but effective approach to object-oriented programming. Python’s elegant syntax and dynamic typing, together with its interpreted nature, make it an ideal language for scripting and rapid application development in many areas on most platforms.
The Python interpreter and the extensive standard library are freely available in source or binary form for all major platforms from the Python Web site, http://www.python.org/,
Programs written in Python are typically much shorter than equivalent C, C++, or Java programs.
- express complex operations
- statement grouping is done by indentation
- no variable or argument declarations
Hello World in Python
# hello-world.py
print 'hello world'
Python is strongly typed
types are enforced
dynamically, implicitly typed
you don’t have to declare variables
case sensitive
var and VAR are two different variables
object-oriented
everything is an object
Getting help
Help in Python is always available right in the interpreter. If you want to know how an object works, all you have to do is call
help(
dir() shows you all the object’s methods
>>> help(5)
Help on int object:
(etc etc)
>>> dir(5)
['__abs__', '__add__', ...]
>>> abs.__doc__
'abs(number) -> number\n\nReturn the absolute value of the argument.'
Python has no mandatory statement termination characters
blocks are specified by indentation. Indent to begin a block, dedent to end one.
Statements that expect an indentation level end in a colon (:).
Comments start with the pound (#) sign and are single-line, multi-line strings are used for multi-line comments.
Values are assigned (in fact, objects are bound to names) with the equals sign (”=”), and
equality testing is done using two equals signs (”==“).
You can increment/decrement values using the += and -= operators respectively by the right-hand amount. This works on many datatypes, strings included.
You can also use multiple variables on one line. For example:
>>> myvar = 3
>>> myvar += 2
>>> myvar
5
>>> myvar -= 1
>>> myvar
4
"""This is a multiline comment.
The following lines concatenate the two strings."""
>>> mystring = "Hello"
>>> mystring += " world."
>>> print mystring
Hello world.
The data structures available in python are lists, tuples and dictionaries.
Lists are like one-dimensional arrays and you can also have lists of other lists
dictionaries are associative arrays also known as hash tables
tuples are immutable one-dimensional arrays
Python “arrays” can be of any type, so you can mix e.g. integers, strings, etc in lists/dictionaries/tuples.
The index of the first item in all array types is 0.
Negative numbers count from the end towards the beginning, -1 is the last item.
Variables can point to functions. The usage is as follows:
>>> sample = [1, ["another", "list"], ("a", "tuple")]
>>> mylist = ["List item 1", 2, 3.14]
>>> mylist[0] = "List item 1 again"
>>> mylist[-1] = 3.14
>>> mydict = {"Key 1": "Value 1", 2: 3, "pi": 3.14}
>>> mydict["pi"] = 3.15
>>> mytuple = (1, 2, 3)
>>> myfunction = len
>>> print myfunction(mylist)
3
You can access array ranges using a colon (:).
Leaving the start index empty assumes the first item,
leaving the end index assumes the last item. Negative indexes count from the last item backwards (thus -1 is the last item) like so:
>>> mylist = ["List item 1", 2, 3.14]
>>> print mylist[:]
['List item 1', 2, 3.1400000000000001]
>>> print mylist[0:2]
['List item 1', 2]
>>> print mylist[-3:-1]
['List item 1', 2]
>>> print mylist[1:]
[2, 3.14]
Strings can use either single or double quotation marks, and you can have quotation marks of one kind inside a string that uses the other kind
“He said ‘hello’.” is valid
Multiline strings are enclosed in triple double (or single) quotes (”“”).
Python supports Unicode , using the syntax u“This is a unicode string”.
To fill a string with values, you use the % (modulo) operator and a tuple.
Each %s gets replaced with an item from the tuple, left to right, and you can also use dictionary substitutions, like so:
>>>print "Name: %s\nNumber: %s\nString: %s" % (myclass.name, 3, 3 * "-")
Name: Poromenos
Number: 3
String: ---
strString = """This is
a multiline
string."""
# WARNING: Watch out for the trailing s in "%(key)s".
>>> print "This %(verb)s a %(noun)s." % {"noun": "test", "verb": "is"}
This is a test.
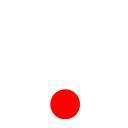