import java.applet.*;
import java.awt.*;
import java.awt.event.*;public class ClickButton extends Applet implements ActionListener{
// declare a textfield and Button
TextField text1;
Button button1;
// initialise the applet
public void init(){
// set the textfield to 30 characters
text1 = new TextField(30);
// add the textfield to the applet
add(text1);
// initialise button and set its label
button1 = new Button(" click me");
// add button to the applet
add(button1);
// set the actionlistener to the button
button1.addActionListener(this);
}
// methods needed for actionlistener Interface
public void actionPerformed(ActionEvent e){
// declare and initialise a string to be shown
String message = " You clicked the button. woo hoo";
// if the source of the action is the button
// ie if the button is clicked
if(e.getSource() == button1){
// set the text of the textfield to our message
text1.setText(message);
}
}
}
And here is the html code to run it, just as a reminder.
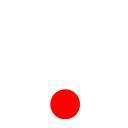