Arrays are lists of similar objects or datatypes. they are ordered in a numbered sequence starting from 0 until the end or last item in the array. Here is an array of integers (int's). Note the format.
{12, 7, 32, 15, 113, 0, 7}
Here is how they will be stored with their number in the list :
index | 0 | 1 | 2 | 3 | 4 | 5 | 6 |
---|---|---|---|---|---|---|---|
value | 12 | 7 | 32 | 15 | 113 | 0 | 7 |
The array items' number in the list is called its index. To get the value of an array item or element you give its index inside [ ] square brackets after the array's name. We could call the above array scoreArray.
Declaration of Arrays
We can declare arrays in Java in 2 ways:
int scoreArray[] ;This declaration tells the compilers what type of variables (datatype) the array contains. No actual array is created until the keyword NEW is invoked or used. e.g.
int[] scoreArray ;
int [] scoreArray ;
scoreArray = new int[7] ;
The 2 lines above put an array of 7 ints into the array called scoreArray and they are all given a default value of 0 .
An array can be declared, constructed, and initialized in a single statement . e.g.
int[] scoreArray = {12, 7, 32, 15, 113, 0, 7 } ;
Note the use of the { } curly brackets and commas between the elements of the array.
Calling an Array Element
To retrieve an element in an array we simply put its index number in square brakets [] after the array name. e.g. scoreArray[0] ;
Exercise:
- what does scoreArray[0] equal ?
- What is the value of scoreArray[5] ?
Remember - arrays start at index 0 . So, the 5th element in an array will be arrayName[4] .
Here is a formula:
nth Element => arrayName[n-1]
Changing Java Array values
If you want to change an array element value, just go something like this :
scoreArray[3] = 56 ;
scoreArray[1] = 27 ;
To put an array element into another variable , just do something like this :
int myScore ;
myScore = scoreArray[4] ;
If you printed out myScore(printLine(myScore) ; ) , it would print 113.
Lets write a program after all that theorizing !
void main() {
// declare , initialize array
int[] scoreArray = {12, 7, 32, 15, 113, 0, 7 } ;
// print scores
for(int i = 0; i<7 ; i++ )
{
printLine("Score "+ i + " = " + scoreArray[i]);
}
}
Java Problem :
You have been given the job by the ACB to write a program to enter the scores for the Australian cricket teams one day match scores.
Output the scores and the Runs Per Over. A One Day Game has 50 overs.
It could have an output like this :
Player 1 : 23
Player 2 : 66
...
Player 11 : 7
Runs Per Over = 4.03
Lets first write out the steps that our program will take, in plain English .
- Declare array of ints containing 11 elements
- Loop through these items to read each score- input
- Add each score to a total
- Calculate the Runs Per Over
- Print out the scores.
- Print out the Runs Per Over
What we have just done is write the program's
ALGORITHM
.
An Algorithm is the series of steps written in plain English that would make our program run successfully or accomplish its task.
Lets write it ! You have a go on your own first.
...
What did you get ?
..
...
When you have done it, try to read in and output each cricketer's name instead of Player 3
e.g.
Calculate the Average score for the team.
And print out those above it and those below the average.
Exercise:
- Are these statements legal ?
a. int[ ] numberArray[ ] ;
b. String[ ] name ;
c. double price[ ] ;
price = new double[12] ;
- What are the default initialization values for int, double, String , boolean ?
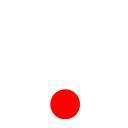