The bubble sort gets its name because as elements are sorted they gradually "bubble" (or rise) to their proper positions, like bubbles rising in a glass of soda. A bubble sort repeatedly compares adjacent elements of an array, starting with the first and second elements, and swapping them if they are out of order. After the first and second elements are compared, the second and third elements are compared, and swapped if they are out of order.
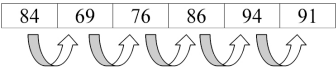
The bubble sort is an easy algorithm to program, but it is slower than many other sorts. With a bubble sort, it is always necessary to make one final "pass" through the array to check to see that no swaps are made to ensure that the process is finished. In actuality, the process is finished before this last pass is made.
// Bubble Sort Method for Descending Order
public static void bubble_sort( int [ ] num )
{
int j;
boolean flag = true; // set flag to true to begin initial pass
int temp; //holding variablewhile ( flag )
{
flag= false; //set flag to false awaiting a possible swap
for( j=0; j < num.length -1; j++ )
{
if ( num[ j ] < num[j+1] ) // change to > for ascending sort
{
temp = num[ j ]; //swap elements
num[ j ] = num[ j+1 ];
num[ j+1 ] = temp;
flag = true; //indicates that a swap occurred
}
}
}
}
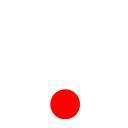