Sorting means to put data in order;
either numerically or alphabetically.
There are many times when it is necessary to put an array in order from highest to lowest (descending) or vice versa (ascending).
Because sorting arrays requires exchanging values, computers must use a special technique to swap the positions of array elements so as not to lose any elements.
Suppose that score[1] = 10 and score[2] = 8 and you want to exchange their values so that score[1] = 8 and score[2] = 10.
You can NOT just do this:
score[1] = score[2];
score[2] = score[1]; // Does NOT Work!!
In the first line, the value of score[1] is erased and replaced with score[2].
The result is that both score[1] and score[2] now have the same value.
You lost the original value that was in score[1].
A temporary holding variable is needed for swapping values.
In order to swap two values, you must use a third variable to temporarily hold the value you do not want to lose:
//swapping variables
temp = score[1]; // holding variable
score[1] = score[2];
score[2] = temp;
This process successfully exchanges the values of the two variables.
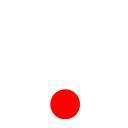