Arrays are passed-by-reference. Passing-by-reference means that when an array is passed as an argument, its memory address location is actually passed, referred to as its "reference".
In this way, the contents of an array CAN be changed inside of a method, since we are dealing directly with the actual array and not with a copy of the array.
int [ ] num = {1, 2, 3};
testingArray(num); //Method call
System.out.println("num[0] = " + num[0] + "\n num[1] = " + num[1] + "\n num[2] =" + num[2]);
. . .//Method for testing
public static void testingArray(int[ ] value)
{
value[0] = 4;
value[1] = 5;
value[2] = 6;
}
Output:
num[0] = 4
num[1] = 5
num[2] = 6
(The values in the array have been changed.
Notice that nothing was "returned".)
Example: Fill an array with 10 integer values. Pass the array to a method that will add up the values and return the sum. (In this example, no original data in the array was altered. The array information was simply used to find the sum.)
import java.io.*;
import BreezyGUI.*;public class FindSum
{
public static void main (String [ ] args)
{
int [ ] number = new int [ 10]; // instantiate the array
int i;
int sum=0;for ( i = 0; i < 10; i++ ) // fill the array
number[ i ] = Console.readInt("Enter number: " );int sum = find_sum(number); // invoke the method
System.out.println("The sum is" +sum + ".");
}public static int find_sum(int [ ] value) //method definition to find sum
{
int i, total = 0;
for(i=0; i<10; i++)
{
total = total + value[ i ];
}return (total);
}
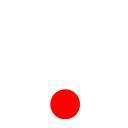