The return value can be a constant, a variable, or an expression. The only requirement is that the return value be of the type specified in the method definition.
//Demo Program for Methods
import java.io.*;
import BreezyGUI.*;public class method1test
{
public static void main(String[ ] args)
{
greeting(5); //first method call
int response;
response = getNumber(); //second method call
greeting (response); //first method call again
}
//First Method for greeting
public static void greeting(int x)
{
int i;
for(i = 0; i < x; i++)
{
System.out.print("Hi ");
}
System.out.println( );
}//Second method which returns a number
public static int getNumber( )
{
int number;
do
{
number = Console.readInt("\nPlease enter value(1-10): ");
}
while ((number < 1) || (number > 10)); //error trap
return number; //return the number to main
}
}
Output:
Hi Hi Hi Hi Hi
Please enter value(1-10): 5
Hi Hi Hi Hi Hi
-----------------
takes arguments AND returns a value.
//Demo Program for Methods
import java.io.*;
import BreezyGUI.*;public class method1test
{
public static void main(String[ ] args)
{
int test1 = 12, test2 = 10;
// notice in this next line the method called min
System.out.println("The minimum of " + test1 + " and " + test2
+ " is " + min(test1,test2) + ".");
}//Method to find minimum
// This method needs to receive two integers and returns one integer.
public static int min(int x, int y)
{
int minimum; // local variable
if (x < y)
minimum = x;
else
minimum = y;
return (minimum); // return value to main
}
}
Output:
The minimum of 12 and 10 is 10.
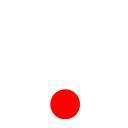