Random Number Generation
Computers can be used to simulate the generation of random numbers with the use of the random( ) method. This random generation is referred to as a pseudo-random generation. These created values are not truly "random" because a seeded mathematical formula is used to generate the values.
Remember that the random( ) method will return a value greater than or equal to zero and less than 1. But don't expect values necessarily like .5 or .25. The following line of code:
double randomNumber = Math.random( );
will most likely generate a number such as 0.7342789374658514. Yeek!
When you think of random numbers for a game or an activity, you are probably thinking of random "integers". Let's do a little adjusting to the random( ) method to see if we can create random integers.
Generating random integers within a specified range:
In order to produce random "integer" values within a specified range, you need to manipulate the random( ) method.
Obviously, we will need to cast our value to force it to become an integer.
The formula is:
int number = (int) (a + Math.random( ) * (b - a + 1));
a = the first number in your range
b = the last number in your range
(b - a + 1 ) is the number of terms in your range.
(range computed by largest value - smallest value + 1)
Examples:
• random integers in the interval from 1 to 10 inclusive.
int number = (int) (1 + Math.random( ) * 10);
• random integers in the interval from 22 to 54 inclusive.
int number = (int) (1 + Math.random( ) * 33);
note: the number of possible integers from 22 to 54 inclusive is 33
b - a + 1 = 54 - 22 + 1 = 33
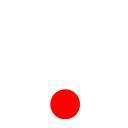