While the Math class is quite extensive, we will limit our attention to the methods listed below.
Method Purpose
abs(int x)
Returns the absolute value of an integer x.
abs(double x)
Returns the absolute value of a double x
pow(double base, double exponent)
Returns the base raised to the exponent.
round(double x)
Returns x rounded to the nearest whole number. Returned value must be cast to an int before assignment to an int variable.
max(int a, int b)
Returns the greater of a and b.
min(int a, int b)
Returns the lesser of a and b.
double sqrt(double x)
Returns the square root of x.
random( )
Returns a random number greater than or equal to 0.0 and less than 1.0.
These methods are used by invoking the name of the class (Math), followed by a dot (.), followed by the name of the method and any needed parameters.
SYNTAX: radius = Math.sqrt(area/Math.PI));
More Examples:
int ans1;
double ans2;
ans1 = Math.abs(-4); //ans1 equals 4
ans1 = Math.abs(-3.6); //ans1 equals 3.6
ans2 = Math.pow(2.0,3.0); //ans2 equals 8.0
ans2 = Math.pow(25.0, 0.5); //ans2 equals 5.0
ans1 = Math.max(30,50); //ans1 equals 50
ans1 = Math.min(30,50); //ans1 equals 30
ans1 = (int) Math.round(5.66); //ans1 equals 6
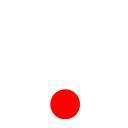