To create a GUI style window, you will add control items to your GBFrame such as input/output boxes (called fields), labels, buttons and more.
You control the layout of a window by imagining a grid superimposed over the window. The grid can contain as many cells as needed.
While most controls occupy only one cell, it is possible for a control to span several cells. In this window, the "Compute Ounces" button spans cells (3,1) and (3,2).
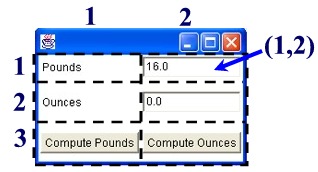
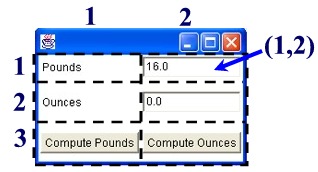
While most controls occupy only one cell, it is possible for a control to span several cells. In this window, the "Compute Ounces" button spans cells (3,1) and (3,2).
//Sample Program
//Using BreezyGUI
import java.awt.*;
import BreezyGUI.*;
public class OuncesPounds extends GBFrame
{
Label poundsLabel = addLabel ("Pounds",1,1,1,1);
Label ouncesLabel = addLabel ("Ounces",2,1,1,1);
DoubleField poundsField = addDoubleField (0 ,1,2,1,1);
DoubleField ouncesField = addDoubleField (0 ,2,2,1,1);
Button poundsButton = addButton ("Compute Pounds",3,1,1,1);
Button ouncesButton = addButton ("Compute Ounces", 3,2,1,1);
public void buttonClicked (Button buttonObj)
{
double pounds, ounces;
if (buttonObj == ouncesButton)
{
pounds = poundsField.getNumber();
ounces = 16 * pounds;
ouncesField.setNumber(ounces);
ouncesField.setPrecision(2);
}
else
{
ounces = ouncesField.getNumber();
pounds=ounces/16;
poundsField.setNumber(pounds);
poundsField.setPrecision(2);
}
}
public static void main (String[ ] args)
{
Frame frm = new OuncesPounds ();
frm.setSize (250, 150);
frm.setVisible (true);
}
}
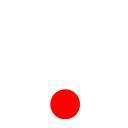