It was noted previously that we will not be using a minus sign (-) to represent negative numbers. We would like to represent our binary numbers with only two symbols, 0 and 1. There are a few ways to represent negative binary numbers. The simplest of these methods is called ones complement, where the sign of a binary number is changed by simply toggling each bit (0's become 1's and vice-versa). This has some difficulties, among them the fact that zero can be represented in two different ways (for an eight bit number these would be 0000 0000 and 1111 1111)., we will use a method called two's complement notation which avoids the pitfalls of one's complement, but which is a bit more complicated.
To represent an n bit signed binary number the leftmost bit, has a special significance. The difference between a signed and an unsigned number is given in the table below for an 8 bit number.
The value of bits in signed and unsigned binary numbers
Bit 7
Bit 6
Bit 5
Bit 4
Bit 3
Bit 2
Bit 1
Bit 0
Unsigned
27 = 128
26 = 64
25 = 32
24= 16
23= 8
22 = 4
21 = 2
20 = 1
Signed
-(27) = -128
26 = 64
25 = 32
24 = 16
23 = 8
22 = 4
21= 2
20 = 1
Let's look at how this changes the value of some binary numbers
Binary
Unsigned
Signed
0010 0011
35
35
1010 0011
163
-93
1111 1111
255
-1
1000 0000
128
-128
If Bit 7 is not set (as in the first example) the representation of signed and unsigned numbers is the same. However, when Bit 7 is set, the number is always negative. For this reason Bit 7 is sometimes called the sign bit. Signed numbers are added in the same way as unsigned numbers, the only difference is in the way they are interpreted. This is important for designers of arithmetic circuitry because it means that numbers can be added by the same circuitry regardless of whether or not they are signed.
To form a two's complement number that is negative you simply take the corresponding positive number, invert all the bits, and add 1. The example below illustrated this by forming the number negative 35 as a two's complement integer:
3510 = 0010 00112
invert -> 1101 11002
add 1 -> 1101 11012
So 1101 1101 is our two's complement representation of -35. We can check this by adding up the contributions from the individual bits
1101 11012 = -128 + 64 + 0 + 16 + 8 + 4 + 0 + 1 = -35.
The same procedure (invert and add 1) is used to convert the negative number to its positive equivalent. If we want to know what what number is represented by 1111 1101, we apply the procedure again
? = 1111 11012
invert -> 0000 00102
add 1 -> 0000 00112
Since 0000 0011 represents the number 3, we know that 1111 1101 represents the number -3.
Convert 1101 1101 from binary to decimal
Convert 0010 0010 from binary to decimal
Convert -120 from decimal to binary
In 'C', a signed integer is usually 16 bits. What is the largest positive number that can be represented?
What is the largest negative number that can be represented?
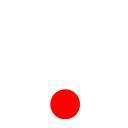