program Inheritance_1;{ ******************** class definitions **********************}
type
Vehicle = object
Wheels : integer;
Weight : real;
constructor Init(In_Wheels : integer; In_Weight : real);
function Get_Wheels : integer;
function Get_Weight : real;
function Wheel_loading : real;
end;Car = object(Vehicle)
Passenger_Load : integer;
constructor Init(In_Wheels : integer;
In_Weight : real;
People : integer);
function Passengers : integer;
end;Truck = Object(Vehicle)
Passenger_Load : integer;
Payload : real;
constructor Init(People : integer;
Max_Load : real;
In_Wheels : integer;
In_Weight : real);
function Efficiency : real;
function Wheel_Loading :real;
end;{ ********************* class implementations ******************** }
constructor Vehicle.Init(In_Wheels : integer; In_Weight : real);
begin
Wheels := In_Wheels;
Weight := In_Weight;
end;function Vehicle.Get_Wheels : integer;
begin
Get_Wheels := Wheels;
end;function Vehicle.Get_Weight : real;
begin
Get_Weight := Weight;
end;function Vehicle.Wheel_loading : real;
begin
Wheel_Loading := Weight/Wheels;
end;constructor Car.Init(In_Wheels : integer;
In_Weight : real;
People : integer);
begin
Wheels := In_Wheels;
Weight := In_Weight;
Passenger_Load := People;
end;function Car.Passengers : integer;
begin
Passengers := Passenger_Load;
end;constructor Truck.Init(People : integer;
Max_Load : real;
In_Wheels : integer;
In_Weight : real);
begin
Passenger_Load := People;
Payload := Max_Load;
Vehicle.Init(In_Wheels, In_Weight);
end;function Truck.Efficiency : real;
begin
Efficiency := 100.0 * Payload / (Payload + Weight);
end;function Truck.Wheel_Loading : real;
begin
Wheel_Loading := (Weight + Payload)/Wheels;
end;{ ************************ main program ************************** }
var Unicycle : Vehicle;
Sedan : Car;
Semi : Truck;begin
Unicycle.Init(1, 12.0);
Sedan.Init(4, 2100.0, 5);
Semi.Init(1, 25000.0, 18, 5000.0);WriteLn('The unicycle weighs ', Unicycle.Get_Weight:5:1,
' pounds, and has ', Unicycle.Get_Wheels, ' wheel.');WriteLn('The car weighs ', Sedan.Get_Weight:7:1,
' pounds, and carries ', Sedan.Passengers,
' passengers.');WriteLn('The semi has a wheel loading of ', Semi.Wheel_Loading:8:1,
' pounds per tire,');
WriteLn(' and has an efficiency of ', Semi.Efficiency:5:1,
' percent.');with Semi do
begin
WriteLn('The semi has a wheel loading of ', Wheel_Loading:8:1,
' pounds per tire,');
WriteLn(' and has an efficiency of ', Efficiency:5:1,
' percent.');
end;
end.
Result of execution
The unicycle weighs 12.0 pounds, and has 1 wheel.
The car weighs 2100.0 pounds, and carries 5 passengers.
The semi has a wheel loading of 1666.7 pounds per tire,
and has an efficiency of 83.3 percent.
The semi has a wheel loading of 1666.7 pounds per tire,
and has an efficiency of 83.3 percent.
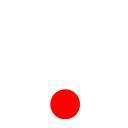