WHAT IS A DATA TYPE?
A type in Pascal, and in several other popular programming languages, defines a variable in such a way that it defines a range of values which the variable is capable of storing, and it also defines a set of operations that are permissible to be performed on variables of that type.
TURBO Pascal has eight basic data types
integer Whole numbers from -32768 to 32767
byte The integers from 0 to 255
real Floating point numbers from 1E-38 to 1E+38
boolean Can only have the value TRUE or FALSE
char Any character in the ASCII character set
shortint The integers from -128 to 127
word The integers from 0 to 65535
longint The integers from -2147483648 to 2147483647
single Real type with 7 significant digits
double Real type with 15 significant digits
extended Real type with 19 significant digits
comp The integers from about -10E18 to 10E18
OUR FIRST VARIABLES
The integers are by far the easiest to understand so we will start with a simple program that uses some integers.
var
Immediately following the program statement is another reserved word, var. This reserved word is used to define a variable before it can be used anywhere in the program.
There is an unbroken rule of Pascal that states "Nothing can be used until it is defined." The compiler will complain by indicating a compilation error if you try to use a variable without properly defining it.
FIRST ARITHMETIC
Now that we have three variables defined as integer type variables, we are free to use them in a program in any way we desire as long as we use them properly. If we tried to assign a real value to X, the compiler will generate an error, and prevent a garbage output. Observe the start of the main body of the program. There are three statements assigning values to X, Y, and Count. A fine point of mathematics would state that Count is only equal to the value of X+Y until one of them is modified,
The first three statements give X the value of 12, Y the value of 13, and Count the value of 12 + 13 or 25. In order to get those values out of the computer,
Multiple outputs can be handled within one Writeln if the fields are separated by a comma. To output a variable, simply write the variable's name in the output field.
BOOLEAN VARIABLES
boolean type variable, which is only allowed to take on two different values, TRUE or FALSE. This variable is used for loop controls, end of file indicators or any other TRUE or FALSE conditions in the program. Variables can be compared to determine a boolean value. A complete list of the relational operators available with Pascal is given in the following list.
= equal to
<> not equal to
> greater than
< less than
>= greater than or equal to
<= less than or equal to
These operators can be used to compare any of the simple types of data including integer, char, byte, and real type variables or constants, and they can be used to compare boolean variables.
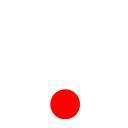