Procedures accept data or variables when they are executed. Functions also accept data, but have the ability to return a value to the procedure or program which requests it. Functions are used to perform mathematical tasks like factorial calculations.
A function
- begins with the keyword function
- is similar in structure to a procedure
- somewhere inside the code associated with the function, a value is assigned to the function name
- a function is used on the righthand side of an expression
- can only return a simple data type
The actual heading of a function differs slightly than that of a procedure. Its format is,
function Function_name (variable declarations) : return_data_type;After the parenthesis which declare those variables accepted by the function, the return data type (preceeded by a colon) is declared.
The following line demonstrates how to call the function,
function ADD_TWO ( value1, value2 : integer ) : integer;
begin
ADD_TWO := value1 + value2
end;
thus, when ADD_TWO is executed, it equates to the value assigned to its name (in this case 30), which is then assigned to result.
result := ADD_TWO( 10, 20 );
SELF TEST
Determine the output of the following program
program function_time (input, output);
const maxsize = 80;
type line = packed array[1..maxsize] of char;function COUNTLETTERS ( words : line) : integer; {returns an integer}
var loop_count : integer; {local variable}
begin
loop_count := 1;
while (words[loop_count] <> '.') and (loop_count <= maxsize) do
loop_count := loop_count + 1;
COUNTLETTERS := loop_count - 1
end;var oneline : line;
letters : integer;
begin
writeln('Please enter in a sentence terminated with a .');
readln( oneline );
letters := COUNTLETTERS( oneline );
writeln('There are ',letters,' letters in that sentence.')
end.
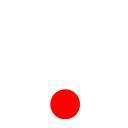