The most common loop in Pascal is the FOR loop. The statement inside the for block is executed a number of times depending on the control condition. The format's for the FOR command is,
FOR var_name := initial_value TO final_value DO program_statement;You must not change the value of the control variable (var_name) inside the loop. The following program illustrates the for statement.FOR var_name := initial_value TO final_value DO
begin
program_statement; {to execute more than one statement in a for }
program_statement; {loop, you group them using the begin and }
program_statement {end statements }
end; {semi-colon here depends upon next keyword }FOR var_name := initial_value DOWNTO final_value DO program_statement;
CLASS EXERCISE
program CELCIUS_TABLE ( output );
var celcius : integer; farenhiet : real;
begin
writeln('Degree''s Celcius Degree''s Farenhiet');
for celcius := 1 to 20 do
begin
farenhiet := ( 9 / 5 ) * celcius + 32;
writeln( celcius:8, ' ',farenhiet:16:2 )
end
end.
What is the resultant output when this program is run.
program FOR_TEST ( output );
var s, j, k, i, l : integer;
begin
s := 0;
for j:= 1 to 5 do
begin
write( j );
s := s + j
end;
writeln( s );
for k := 0 to 1 do write( k );
for i := 10 downto 1 do writeln( i );
j := 3; k := 8; l := 2;
for i := j to k do writeln( i + l )
end.
Class Exercise .. Output of program FOR_TEST is,
1234515
0110
9
8
7
6
5
4
3
2
1
5
6
7
8
9
10
---------------
For the first twenty values of farenhiet, print out the equivalent degree in celcuis (Use a tabular format, with appropiate headings). [C = ( 5 / 9 ) * Farenhiet - 32]Use the statement writeln('<14>'); to clear the screen.
PROGRAM NINE Table of 1 to 20 Celcius
program PROG9 (output);
var farenhiet : real;
celcius : integer;
begin
writeln('<14>'); {clear screen on DG machine}
writeln('Degree''s Celcius Degree''s Farenhiet');
for celcius := 1 to 20 do
begin
farenhiet := ( 9 / 5 ) * celcius + 32;
writeln( celcius:8, ' ', farenhiet:16:2 )
end
end.
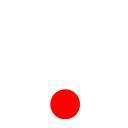