The IF statement can also include an ELSE statement, which specifies the statement (or block or group of statements) to be executed when the condition associated with the IF statement is false. Rewriting the previous program using an IF THEN ELSE statement,
There are times when you want to execute more than one statement when a condition is true (or false for that matter). Pascal makes provison for this by allowing you to group blocks of code together by the use of the begin and end keywords. Consider the following portion of code,
{ Program example demonstrating IF THEN ELSE statement }
program IF_ELSE_DEMO (input, output);
var number, guess : integer;
begin
number := 2;
writeln('Guess a number between 1 and 10');
readln( guess );
if number = guess then
writeln('You guessed correctly. Good on you!')
else
writeln('Sorry, you guessed wrong.')
end.
if number = guess then
begin
writeln('You guessed correctly. Good on you!');
writeln('It may have been a lucky guess though')
end {no semi-colon if followed by an else }
else
begin
writeln('Sorry, you guessed wrong.');
writeln('Better luck next time')
end; {semi-colon depends on next keyword }
What is displayed when the following program is executed?
program IF_THEN_ELSE_TEST (output);
var a, b, c, d : integer;
begin
a := 5; b := 3; c := 99; d := 5;
if a > 6 then writeln('A');
if a > b then writeln('B');
if b = c then
begin
writeln('C');
writeln('D')
end;
if b <> c then writeln('E') else writeln('F');
if a >= c then writeln('G') else writeln('H');
if a <= d then
begin
writeln('I');
writeln('J')
end
end.AnswerClass Exercise .. Program output of IF_THEN_ELSE_TEST is...
B
E
H
I
J
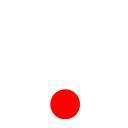