The program which follows reads two numbers from the keyboard, assigns them to the specified variables, then prints them to the console screen.
When run, the program will display the message
program READDEMO (input, output);
var numb1, numb2 : integer;
begin
writeln('Please enter two numbers separated by a space');
read( numb1 );
read( numb2 );
writeln;
writeln('Numb1 is ', numb1 , ' Numb2 is ', numb2 )
end.
then wait for you to enter in the two numbers. If you typed the two numbers, then pressed the return key, eg,
Please enter two numbers separated by a space
then the program will accept the two numbers, assign the value 237 to numb1 and the value 64 to numb2, then continue and finally print
237 64
Numb1 is 237 Numb2 is 64
Differences between READ and READLN
The readln statement discards all other values on the same line, but read does not. In the previous program, replacing the read statements with readln and using the same input, the program would assign 237 to numb1, discard the value 64, and wait for the user to enter in another value which it would then assign to numb2.
The
SELF TEST on READ
Assuming that we made the following declaration
and that the user types
var C1, C2, C3, C4, C5, C6 : char;
ABCDE
When variables are displayed, our version of Pascal assigns a specified number of character spaces (called a field width) to display them. The field widths for the various data types are,
INTEGER - Number of digits + 1 { or +2 if negative }
CHAR - 1 for each character
REAL - 12
BOOLEAN - 4 if true, 5 if false
Often, the allotted field size is too big for the majority of display output. Pascal provides a way in which the programmer can specify the field size for each output.
The display output will be as follows,
writeln('WOW':10,'MOM!':10,'Hi there.');
Note that to specify the field width of text or a particular variable, use a colon (:) followed by the field size.
WOW.......MOM!......Hi there. ... indicates a space.
'text string':fieldsize, variable:fieldsize
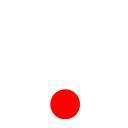