The input parts.
When we ask computer to receive input from users, we need something to store the input before it is being processed. That was variable.
We need it to corporate our program. There are various types of variable and with various range too. Here are some frequently used variable types :
Type name | Range | Type |
---|---|---|
Shortint | -128 to +127 | integer |
Byte | 0 to 255 | integer |
Integer | -32768 to +32767 | integer |
Word | 0 to 65535 | integer |
Longint | -2146473648 to +2146473647 | integer |
Real | -??????? to +??????? | fractional |
String | up to 255 letters | non-numeric |
Char | 1 letter only | non-numeric |
There are many more...
How to declare our variables ?
Example :
var
MyAge : Integer;
Comments : String;
To assign variables with values, you need to write this syntax :
var_name := value;
This should be written anywhere within begin ... end block, in appropriate location.
Example :
var
MyAge : Byte;
Comments : String;
begin
MyAge:=19;
Comments:='Hi, there ! I'm learning Pascal';
Writeln('I am ',MyAge,' years old');
Writeln(Comments);
end.
How to get the user's input ?
The difference is that in Read or Readln,
we cannot write anything on screen but to get user's input.
Example :
var
MyAge : Byte;
Comments : String;
begin
Write('How old are you ? '); Readln(MyAge);
Write('Give us comments: '); Readln(Comments);
Writeln;
Writeln('I am ',MyAge,' years old');
Writeln(Comments);
end.
Now, you know how to get user's input.
The value on that question is actually stored in MyAge.
MyAge is a Integer variable. So it only accept integer values between -127 to +127.
If you want computer to accept fractional values in MyAge,
try using Real instead of Integer.
Writeln('I am ',MyAge,' years old');
to :
Writeln('I am ',MyAge:2:4,' years old');
What does it mean ? Why some :2:4 attached to MyAge ? For real numbers, it means : Show 2 place before decimal point and 4 place after decimal point So, when we enter 23.5 for MyAge, it will output :
I am 23.5000 years oldTry changing :2:4 with other values. Be creative !
Can we apply it to the string ? No ! But it only accepts just :x. It means that 'I only grant this variable x place on the screen to express its value'. Suppose you change :
Writeln(Comments);
to
Writeln(Comments:5);
Try writing long comments when the program is running. See what happens. If you enter Programming as the Comment, you will see output :
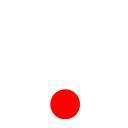