Turbo Pascal is a complete development system that includes a compiler and an Integrated Development Environment (IDE) for the Pascal programming language running mainly on MS-DOS, developed by Borland under Philippe Kahn's leadership.
Download Turbo Pascal 5.5 (963 K)
The name Borland Pascal was generally reserved for the high end packages (with more libraries and standard library source code) while the original cheap and widely known version was sold as Turbo Pascal. The name Borland Pascal is also used more generically for Borland's dialect of Pascal.
Borland has released three versions of Turbo Pascal for free: versions 1.0, 3.02 and 5.5.
The name Borland Pascal was generally reserved for the high end packages (with more libraries and standard library source code) while the original cheap and widely known version was sold as Turbo Pascal. The name Borland Pascal is also used more generically for Borland's dialect of Pascal.
Borland has released three versions of Turbo Pascal for free: versions 1.0, 3.02 and 5.5.
A Simple Program
program HelloWorld(output);
begin
writeln('Hello, World!')
end.
Data structures
Pascal's simple data types are real, integer, character, boolean and enumerations, a new type constructor introduced with Pascal:
var
r: Real;
i: Integer;
c: Char;
b: Boolean;
e: (apple, pear, banana, orange, lemon);
Subranges of any ordinal type (any simple type except real) can be made:
var
x: 1..10;
y: 'a'..'z';
z: pear..orange;
In contrast with other programming languages Pascal supports set type:
var
set1: set of 1..10;
set2: set of 'a'..'z';
set3: set of pear..orange;
A set is fundamental concept for modern mathematics,
and many algorithms are defined with sets usage.
So, an implementation of such algorithm is very suitable for Pascal.
Also, set's operations may be realized faster.
For example, for many Pascal compilers:
if i in [5..10] then
...
is faster, than
if (i>4) and (i<11) then
...
Types can be defined from other types using type declarations:
type
x = Integer;
y = x;
...
Further, complex types can be constructed from simple types:
type
a = Array [1..10] of Integer;
b = record
x: Integer;
y: Char
end;
c = File of a;
Control structures
Pascal is a structured programming language,
meaning that the flow of control is structured into standard statements,
ideally without 'go to' commands.
while a <> b do writeln('Waiting');
if a > b then
writeln('Condition met')
else
writeln('Condition false');
for i := 1 to 10 do writeln('Iteration: ', i:1);
repeat a := a + 1 until a = 10;
Procedures and functions
Pascal structures programs into procedures and functions.
program mine(output);
var i : integer;
procedure print(var j: integer);
function next(k: integer): integer;
begin
next := k + 1
end;
begin
writeln('The total is: ', j);
j := next(j)
end;
begin
i := 1;
while i <= 10 do print(i)
end.
Procedures and functions can nest to any depth, and the 'program' construct is the logical outermost block.
Each procedure or function can have its own declarations of goto labels, constants, types, variables, and other procedures and functions, which must all be in that order. This ordering requirement was originally intended to allow efficient single-pass compilation. However, in some dialects the strict ordering requirement of declaration sections is not required.
Pointers
Pascal supports the use of pointers:
type
a = ^b;
b = record
x: Integer;
y: Char;
z: a
end;
var
pointer_to_b: a;
Here the variable pointer_to_b is a pointer to the data type b, a record. Pointers can be used before they are declared. This is an exception to the rule that things must be declared before they are used. To create a new record and assign the values 10 and A to the fields a and b in the record, the commands would be;
new(pointer_to_b);
pointer_to_b^.x := 10;
pointer_to_b^.y := 'A';
pointer_to_b^.z := nil;
...
This could also be done using the with statement, as follows
new(pointer_to_b);
with pointer_to_b^ do
begin
x := 10;
y := 'A';
z := nil
end;
...
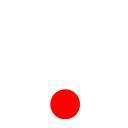