Conversion Functions
To get any numbers contained in a string
Val(String)
The following code gets any numbers contained in String, and puts it into a textbox
Dim sNum As String
sNum = "Year: 2000, date: 12"
Text1.Text = Val(sNum)
convert numbers to different types.
For example, the Int function converts any number to a Integer (whole number). The following code will display a message box with 123 in it.
Dim fNum As Single
fNum = 1234.90
Msgbox Int(fNum)
You can also use the following commands:
Int - Converts a number to a Integer
CLng - Converts a number to a Long
Hex - Converts a number to Hex
Random Numbers
You can use Rnd and Randomize to generate random numbers. The Rnd Function uses the following syntax:
Rnd()
However, the Rnd Function returns a random number less than one.
in order to get a whole number, you need to use the Int function. If you need a larger range than 0 or 1, you need to multiply the result.
The following code simulates a dice rolling:
Randomize
Dice1 = Int(5 * Rnd) + 1
Msgbox "You rolled a " & Dice1
the statement Int(5*rnd) generates a number from 0 to 5.
You add one after as you cannot role a 0.
The Int statement rounds up the number from the Rnd function, as the RND function returns a number less than 1.
The Randomize statement initializes the Rnd function. Without this, each time you run your application you will get the same sequence of numbers.
To produce random integers in a given range, use this formula:
Int((upperbound - lowerbound + 1) * Rnd + lowerbound)
Here, upperbound is the highest number in the range, and lowerbound is the lowest number in the range.
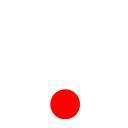