Registry
- GetSetting - Get a value from the Registry
- temp$ = getsetting "TestApp", "SectionName", " KeyName", "defaultvalue"
- GetAllSettings -Returns a list of key settings and their values
- GetAllSettings ( appname,section)
- SaveSetting - Save a value into the Registry
- savesetting " TestApp", SectionName, KeyData
- DeleteSetting - Deletes an entry from the registry
- deletesetting "TestApp", " SectionName", "Keyname"
- deletesetting "TestApp", " SectionName", "Keyname"
Loops and Conditional Decisions
- If ..Then ..Else - Performs code based on the results of a test
- If A5 Then Print "A is a bit number!"
- For...Next - Loops a specified number of times
- For i = 1 to 5: print #1, i: next i
- For Each ... Next - Walks through a collection
- For Each X in Form1.controls: Next X
- While...Wend - Loops until an event is false
- while i 5: i = i +1: wend
- Select Case - Takes an action based on a value of a parameter
- select case i
- case 1 : print "it was a 1"
- case 2 : print "it was a 2"
- end select
- select case i
- Do...Loop - Loops until conditions are met
- do while i 5 : i = i + 1 : loop
- IIF - Returns 1 of two parts, depending on the value of an expression
- result = IIF (testexpression, truepart, falsepart)
- Choose - Selects and returns a value from a list of arguments
- Choose (index, "answer1", "answer2", "answer3")
- With - Executes a series of statements on a single object
- With textbox1
- .Height = 100
- .Width = 500
- End With
- With textbox1
- End - Immediately stops execution of a program
- End
- Stop - Pauses execution of a program (can restart without loss of data)
- Stop
- Switch - Returns a value associated with the first true expression in a list
- result = Switch (testvalue1, answer1, testvalue2, answer2)
- GoTo - Switches execution to a new line in the code
- GoTo Line1
- GoSub ... Return - Switches execution to a new block of code and then returns
- GoSub Line1
- On .. GoSub - Branch to a specific line of code then return at the next Return statement
- On Number GoSub Line1, Line2, Line3
- On .. GoTo - Branch to a specific line of code
- On Number GoTo Line1, Line2, Line3
Special Values
- True - A logical (Boolean) expression. In VB, its value is -1
- X = TRUE
- False - A logical (Boolean expression. In VB, its value is 0
- X = FALSE
- Nothing - Disassociates an object variable from an actual object
- Set X = Nothing
- Null - Indicates that a variable has no valid data
- X = Null
- Empty - Indicates that a variable has not yet been initialized
- X = Empty
Error Handling
Use these VB features to help me figure out what went wrong.
- On Error - Enables an error-handling routine
- On Error GoTo Line2 (if error occurs, go to line2)
- On Error Resume Next (if error occurs, continue executing next line of code)
- On Error Goto 0 (disables error handling)
- On Error GoTo Line2 (if error occurs, go to line2)
- Resume - Used to resume execution after a error-handling routine is finished
- Resume
- Resume Next
- Resume Line1
- Resume
- CVErr - Returns an error type variable containing a user-specific error number
- X = CVError(13)
- Error - Simulates the occurrence of an error
- Error 23
Financial Calculations
- DDB - Returns the depreciation of an asset for a specific time period
- FV - Returns the future value of an annuity
- IPmt - Returns the interest payment of an investment
- IRR - Returns the internal rate of return on a cash flow
- MIRR - Returns a modified internal rate of return on a cash flow
- NPer - Returns a number of periods for an annuity
- NPV - Returns a present value of an investment
- PPmt - Returns the principal payment of an annuity
- PV - Returns the present value of an annuity
- Rate - Returns the interest rate per period for an annuity
- SLN - Returns the straight-line depreciation of an asset
- SYD - Returns the sum-of-years' digits depreciation of an asset
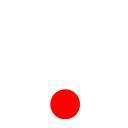