File Handling (Generic)
- Dir - Returns a filename that matches a pattern
- temp $ = Dir ("*.*")
- CurDir - Returns the current directory
- temp$ = CurDir
- MkDir - Creates a directory
- mkdir ( "newdirectoryname" )
- ChDir - Changes the current directory to a new location
- chdir ( "newdirectoryname" )
- ChDrive - Changes the current drive
- ChDirve "A"
- RmDir - Removes the indicated directory
- rmdir ( "directoryname" )
- Freefile - Returns an unused file handle
- i = freefile
- Open - Opens a file for access, locking it from other applications
- open "filename" for input as #1
- Close - Closes a file so that other applications may access it
- close #1
- LOF - Returns the length of a file in bytes
- i = lof ( #1 )
- EOF - Returns a boolean value to indicate if the end of a file has been reached
- statusvariable = eof ( #1 )
- Name As - Renames a file
- name "filename1" as "filename2"
- Kill - Deletes a file
- kill "filename"
- Fileattr - Returns attribute information about a file
- i = int ( tempvariable )
- GetAttr - Returns attributes of a file or directory
- i = GetAttr("c:\windows\temp")
- SetAttr - Sets the attributes of a file
- SetAttr pathname, vbHidden
- Reset - Closes all disk files opened by the OPEN statement
- Reset
- FileDateTime - Returns data file was created or last edited
- FileDateTime ( filename )
- FileLen - Returns length of file in bytes
- FileLen ( filename )
- FileCopy - Copies a file to a new name
- FileCopy sourcefile, destinationfile
- Lock - Controls access to a part or all of a file opened by OPEN
- Lock #1
- UnLock - Restores access to a part or all of a file opended by OPEN
- UnLock #1
- Width # - Set the output line width used by the OPEN statement
- Width #2, 80
File Handling - ASCII-specific
- Line Input - Reads an entire line of ASCII text
- line input #1, tempvariable$
- Write - Puts data in a file, with separators for the data
- write #1, tempvariable$
- Print - Puts data in a file with no separators
- print #1, tempvariable$
- Spc - Used in a print statement to move a number of spaces
- Print #2, var1 spc(15) var2
- Tab - Used in a print statement to move to TAB locations
- Print #2, var1 Tab(20) var2
File Handling - Binary-specific
- Get - Reads data from a file
- get #1, anyvariable
- Put - Puts data into a file
- put #1, anyvariable
- Seek - Moves the current pointer to a defined location in a file
- seek #1, 26
- Input
- input #1, anyvariable
- Loc - Returns current position with an open file
- i = Loc(#2)
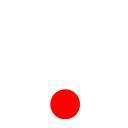