1. Write a C program to compute the sum of the two input values. If the two values are the same, then return triple their sum.
Expected Output:
3
12
Example: Expected Output:
3
12
#include// Include standard input/output library int test(int x, int y); // Declare the function 'test' with two integer parameters int main(void) { // Call the function 'test' with arguments 1 and 2 and print the result printf("%d", test(1, 2)); // Print a newline for formatting printf("\n"); // Call the function 'test' with arguments 2 and 2 and print the result printf("%d", test(2, 2)); } // Function definition for 'test' int test(int x, int y) { // Conditional expression: If x is equal to y, return (x + y) multiplied by 3, otherwise return x + y return x == y ? (x + y) * 3 : x + y; }
2. Write a C program that will take a number as input and find the absolute difference between the input number and 51. If the input number is greater than 51, it will return triple the absolute difference.
#include// Include standard input/output library int test(int n); // Declare the function 'test' with an integer parameter int main(void) { // Call the function 'test' with argument 53 and print the result printf("%d", test(53)); // Print a newline for formatting printf("\n"); // Call the function 'test' with argument 30 and print the result printf("%d", test(30)); // Print a newline for formatting printf("\n"); // Call the function 'test' with argument 51 and print the result printf("%d", test(51)); } // Function definition for 'test' int test(int n) { const int x = 51; // Declare and initialize constant variable 'x' if (n > x) // Check if 'n' is greater than 'x' { return (n - x) * 3; // Return the result of the expression (n - x) multiplied by 3 } return x - n; // Return the result of the expression x minus n }
3. Write a C program that checks two given integers and returns true if at least one of them is 30 or if their sum is 30. In other words, if either of the two integers is 30 or if their sum equals 30, the program will return true.
#include// Include standard input/output library int test(int x, int y); // Declare the function 'test' with two integer parameters int main(void) { // Call the function 'test' with arguments 25 and 5, and print the result printf("%d", test(25, 5)); // Print a newline for formatting printf("\n"); // Call the function 'test' with arguments 20 and 30, and print the result printf("%d", test(20, 30)); // Print a newline for formatting printf("\n"); // Call the function 'test' with arguments 20 and 25, and print the result printf("%d", test(20, 25)); } // Function definition for 'test' int test(int x, int y) { // Return 1 (true) if any of the following conditions are true: // 1. x is equal to 30 // 2. y is equal to 30 // 3. the sum of x and y is equal to 30 return x == 30 || y == 30 || (x + y == 30); }
4. Write a C program to check a given integer and return true if it is within 10 of 100 or 200.
#include// Include standard input/output library #include // Include standard library for absolute value function int test(int x); // Declare the function 'test' with an integer parameter int main(void) { // Call the function 'test' with argument 103 and print the result printf("%d", test(103)); // Print a newline for formatting printf("\n"); // Call the function 'test' with argument 90 and print the result printf("%d", test(90)); // Print a newline for formatting printf("\n"); // Call the function 'test' with argument 89 and print the result printf("%d", test(89)); } // Function definition for 'test' int test(int x) { // Check if the absolute difference between 'x' and 100 is less than or equal to 10, // or if the absolute difference between 'x' and 200 is less than or equal to 10. // Return 1 (true) if either condition is true, otherwise return 0 (false). if (abs(x - 100) <= 10 || abs(x - 200) <= 10) return 1; return 0; }
5. Write a C program that checks two given temperatures and returns true if one temperature is less than 0 and the other is greater than 100, otherwise it returns false.
Expected Output:
#include// Include standard input/output library #include // Include standard library for absolute value function int test(int temp1, int temp2); // Declare the function 'test' with two integer parameters int main(void) { // Call the function 'test' with arguments 120 and -1, and print the result printf("%d", test(120, -1)); // Print a newline for formatting printf("\n"); // Call the function 'test' with arguments -1 and 120, and print the result printf("%d", test(-1, 120)); // Print a newline for formatting printf("\n"); // Call the function 'test' with arguments 2 and 120, and print the result printf("%d", test(2, 120)); } // Function definition for 'test' int test(int temp1, int temp2) { // Check if either of the following conditions are true: // 1. temp1 is less than 0 and temp2 is greater than 100 // 2. temp2 is less than 0 and temp1 is greater than 100 // Return 1 (true) if either condition is true, otherwise return 0 (false). return temp1 < 0 && temp2 > 100 || temp2 < 0 && temp1 > 100; }
7. Write a C program to print numbers from 0 to 10 using a for loop.
#includeint main() { // Loop from 0 to 10 (inclusive) for (int i = 0; i <= 10; i++) { printf("%d\n", i); // Print the current value of 'i' } return 0; }
7.Write a C program to print numbers from 0 to 10 and 10 to 0 using two while loops.
#includeint main() { int i = 0; // Initialize the loop counter // First while loop: Print numbers from 0 to 10 printf("Numbers from 0 to 10:\n"); while (i <= 10) { printf("%d ", i); // Print the current value of i i++; // Increment the loop counter } printf("\n"); // Move to the next line for better formatting i = 10; // Reset the loop counter to 10 // Second while loop: Print numbers from 10 to 0 printf("\nNumbers from 10 to 0:\n"); while (i >= 0) { printf("%d ", i); // Print the current value of i i--; // Decrement the loop counter } return 0; // Indicate successful program execution }
8. Write a C program to print even numbers from 0 to 10.
#includeint main() { // Loop from 0 to 10 for (int i = 0; i <= 10; i++) { // Check if the current number is even if (i % 2 == 0) { printf("%d\n", i); // Print the current even number } } return 0; }
9. Write a C program that calculates the sum of numbers from 1 to 10.
#includeint main() { int sum = 0; // Variable to store the sum // Loop from 1 to 10 for (int i = 1; i <= 10; i++) { sum += i; // Add the current number to the sum } // Print the sum printf("The sum of numbers from 1 to 10 is: %d\n", sum); return 0; }
10. Write a C program that calculates the product of numbers from 1 to 10.
#includeint main() { int product = 1; // Variable to store the product // Loop from 1 to 10 for (int i = 1; i <= 10; i++) { product *= i; // Multiply the current number with the product } // Print the product printf("The product of numbers from 1 to 10 is: %d\n", product); return 0; }
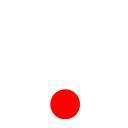