You are given a list of n integers, and your task is to calculate the number of distinct values in the list.
Input: The first input line has an integer n: the number of values.
The second line has n integers x1,x2,…,xn.
Output:
Print one integers: the number of distinct values.
Constraints :
1≤n≤2⋅105 1≤xi≤109Example:
Input:
5 2 3 2 2 3Output:
2
Array based Solution
#include < iostream> using namespace std; int countDistinct(int arr[], int n){ int res = 1; // Pick all elements one by one for (int i = 1; i < n; i++) { int j = 0; for (j = 0; j < i; j++){ if (arr[i] == arr[j]){ break; } // If not counted earlier, then count it if (i == j){ res++; } } return res; } int main(){ int arr[] = { 12, 10, 9, 45, 2, 10, 10, 45 }; int n = sizeof(arr) / sizeof(arr[0]); cout << countDistinct(arr, n); return 0; }
Using vectors
#include < iostream> #include< vector> using namespace std; int main(){ int arr[] = { 12, 10, 9, 45, 2, 10, 10, 45 }; int n = sizeof(arr) / sizeof(arr[0]); vectornums(begin(arr), end(arr)); sort(nums.begin(),nums.end()); int dv=nums[0] ; int dn=1; for (int i = 1; i < nums.size(); i++) { if (dv !=nums[i]) { dn++; dv=nums[i] ; cout << nums[i] << endl; } } return 0; }
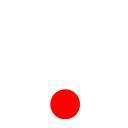