Task :
Find first repeating element in an array of integers
Example:
Take array as input.
Run two loops
The first loop selects every element from the array and second loop traverse ahead and check for the duplicates.
If duplicate is found, print the first repeating integer else print no integer repeated.
Code:
Find first repeating element in an array of integers
Example:
Input: {5, 15, 20, 5, 6, 10, 15, 10} Output: 5# Algorithm
Take array as input.
Run two loops
The first loop selects every element from the array and second loop traverse ahead and check for the duplicates.
If duplicate is found, print the first repeating integer else print no integer repeated.
Code:
#include < iostream> using namespace std; int main(){ int array[100], n, i; cout << "Enter number of elements: "; cin >> n; cout << "\nEnter elements: "; for (i = 0; i < n; i++) cin >> array[i]; cout << "Original array: "; for (int i = 0; i < n; i++) cout << array[i] << " "; // selecting an element for (int i = 0; i < n; i++) //traversing to check repetition for (int j = i + 1; j < n; j++) if (array[i] == array[j]){ cout << "\nFirst repeating integer is " << array[i]; return 0; } cout << "No integer repeated\n"; return 0; }
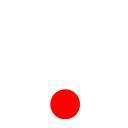