Python provides built-in types for fundamental data types such as numbers, strings, tuples, lists, and dictionaries. You can also create user-defined types, known as classes.
Numbers
Python support integers (plain and long), floating-point numbers, and complex numbers.
Integer numbers
Integer literals can be decimal, octal, or hexadecimal.
A decimal literal is represented by a sequence of digits in which the first digit is nonzero.
To denote an octal literal, use 0 followed by a sequence of octal digits(0 to 7).
To indicate a hexadecimal literal, use 0x followed by a sequence of hexadecimal digits
(0 to 9 and A to F, in either upper- or lowercase). For example:
1, 23, 3493 # Decimal integers
01, 027, 06645 # Octal integers
0x1, 0x17, 0xDA5 # Hexadecimal integers
1L, 23L, 99999333493L # Long decimal integers
01L, 027L, 01351033136165L # Long octal integers
0x1L, 0x17L, 0x17486CBC75L # Long hexadecimal integers
Floating-point numbers
A floating-point literal is represented by a sequence of decimal digits that includes a decimal point (.), an exponent part (an e or E, optionally followed by + or -, followed by one or more digits), or both. The leading character of a floating-point literal cannot be e or E; it may be any digit or a period (.).
For example:
0., 0.0, .0, 1., 1.0, 1e0, 1.e0, 1.0e0
A string literal can be quoted or triple-quoted. A quoted string is a sequence of zero or more characters enclosed in matching quotes, single (') or double ("). For example:
'This is a literal string'
"This is another string"
The two different kinds of quotes function identically; having both allows you to include one kind of quote inside of a string specified with the other kind without needing to escape them with the backslash character (\):
'I\'m a Python fanatic' # a quote can be escaped
"I'm a Python fanatic" # this way is
more readable
String escape sequences
\
\\ Backslash 0x5c
\' Single quote 0x27
" Double quote 0x22
\a Bell 0x07
\b Backspace 0x08
\f Form feed 0x0c
\n Newline 0x0a
A tuple is an ordered sequence of items.
(100, 200, 300) # Tuple with three items
(3.14,) # Tuple with one item
() # Empty tuple
tuple('wow')
This builds a tuple equal to:
('w', 'o', 'w')
Lists
A list is an ordered sequence of items. The items of a list are arbitrary objects and may be of different types.
[42, 3.14, 'hello'] # List with three items
[100] # List with one item
[] # Empty list
list('wow')
This builds a list equal to:
['w', 'o', 'w']
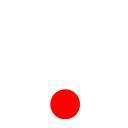