Task:
Separate even and odd numbers from an array of integers. Put all even numbers first, and then odd numbers.
Given:
An array having even and odd integers. We have to segregate even and odd integers and put even numbers first and then odd numbers.
Example:
Initialise two index variables left = 0 and right = n-1.
We have to increment the left index until we see an odd number.
We have to decrement the right index until we see an even number.
If left < right then swap array[left] and array[right].
Code:
Separate even and odd numbers from an array of integers. Put all even numbers first, and then odd numbers.
Given:
An array having even and odd integers. We have to segregate even and odd integers and put even numbers first and then odd numbers.
Example:
Input: {2, 5, 1, 10, 3, 6, 7, 8} Output: 2 8 6 10 3 1 7 5# Algorithm
Initialise two index variables left = 0 and right = n-1.
We have to increment the left index until we see an odd number.
We have to decrement the right index until we see an even number.
If left < right then swap array[left] and array[right].
Code:
#includeusing namespace std; void swap(int * x, int * y){ int t = * x; * x = * y; * y = t; } void segregate(int array[], int n){ int left = 0, right = n - 1; while (left < right){ while (array[left] % 2 == 0 && left < right) left++; while (array[right] % 2 == 1 && left < right) right--; if (left < right){ swap( & array[left], & array[right]); left++; right--; } } } int main(){ int array[100], n, i; cout << "Enter number of elements: "; cin >> n; cout << "\nEnter elements: "; for (i = 0; i < n; i++) cin >> array[i]; cout << "Original array: "; for (int i = 0; i < n; i++) cout << array[i] << " "; segregate(array, n); cout << "\nArray after divided: "; for (int i = 0; i < n; i++) cout << array[i] << " "; return 0; }
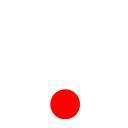